플라이웨이트 패턴 (Flyweight Pattern)
- Coding/Java
- 2023. 4. 2.
반응형
728x90
반응형
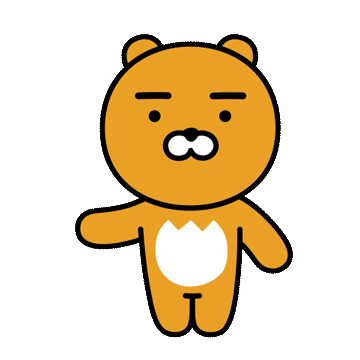
수강완료한 강의 복습해보자
(코딩으로 학습하는 GoF의 디자인 패턴)
플라이웨이트 패턴(Flyweight Pattern)
- 객체를 가볍게 만들어 메모리 사용을 줄이는 패턴.
- 자주 변하는 속성(또는 외적인 속성, extrinsit)과 변하지 않는 속성(또는 내적인 속성, intrinsit)을 분리하고 재사용하여 메모리 사용을 줄일 수 있다.
적용 전 코드 (Before)
Character.java
public class Character {
private char value;
private String color;
private String fontFamily;
private int fontSize;
public Character(char value, String color, String fontFamily, int fontSize) {
this.value = value;
this.color = color;
this.fontFamily = fontFamily;
this.fontSize = fontSize;
}
}
Client.java
public class Client {
public static void main(String[] args) {
Character c1 = new Character('h', "white", "Nanum", 12);
Character c2 = new Character('e', "black", "Nanum", 12);
Character c3 = new Character('l', "white", "Nanum", 12);
Character c4 = new Character('l', "white", "Nanum", 12);
Character c5 = new Character('o', "white", "Nanum", 12);
}
}
변하는 속성인 value, color만 다르고, 변하지 않는 속성인 fontFamily, fontSize가 모두 동일한 객체를 중복 코드로 생성한다.
적용 후 코드 (After)
Font.java
- 변하지않는 속성
- final 변수 선언
- final 클래스 선언 - 상속 불가능
- set 메서드 제공 X (immutable)
public final class Font {
final String family;
final int size;
public Font(String family, int size) {
this.family = family;
this.size = size;
}
public String getFamily() {
return family;
}
public int getSize() {
return size;
}
}
Character.java
- 자주 변하는 속성 value, color를 필드로 갖는다.
import com.designpattern.report._11_flyweight.step2_after.flyweight.Font;
public class Character {
private char value;
private String color;
private Font font;
public Character(char value, String color, Font font) {
this.value = value;
this.color = color;
this.font = font;
}
}
FontFactory.java
import com.designpattern.report._11_flyweight.step2_after.flyweight.Font;
import java.util.HashMap;
import java.util.Map;
public class FontFactory {
private Map<String, Font> cache = new HashMap<>();
/**
* 캐시 처리
* @param font
* @return
*/
public Font getFont(String font) {
if (cache.containsKey(font)) {
return cache.get(font);
} else {
String[] split = font.split(":");
Font newFont = new Font(split[0], Integer.parseInt(split[1]));
cache.put(font, newFont);
return newFont;
}
}
}
1) cache 객체를 사용한다.
만약 기존에 존재하는 key(font)로 요청이 온다면 cache 안의 객체를 리턴해주고, 신규 요청이라면 생성하여 리턴해준다.
Font newFont = new Font(split[0], Integer.parseInt(split[1]));
cache.put(font, newFont);
return newFont;
Client.java
import com.designpattern.report._11_flyweight.step2_after.flyweightFactory.FontFactory;
public class Client {
public static void main(String[] args) {
FontFactory fontFactory = new FontFactory();
// "Nanum", 12 얘를 Font 로 묶음
// 변하지 않는 속성 Font 캐시 처리
Character c1 = new Character('h', "white", fontFactory.getFont("nanum:12"));
Character c2 = new Character('e', "white", fontFactory.getFont("nanum:12"));
Character c3 = new Character('l', "white", fontFactory.getFont("nanum:12"));
}
}
플라이웨이트 적용 전과 비교
Before
public class Client {
public static void main(String[] args) {
Character c1 = new Character('h', "white", "Nanum", 12);
Character c2 = new Character('e', "black", "Nanum", 12);
Character c3 = new Character('l', "white", "Nanum", 12);
Character c4 = new Character('l', "white", "Nanum", 12);
Character c5 = new Character('o', "white", "Nanum", 12);
}
}
After
import com.designpattern.report._11_flyweight.step2_after.flyweightFactory.FontFactory;
public class Client {
public static void main(String[] args) {
FontFactory fontFactory = new FontFactory();
// "Nanum", 12 얘를 Font 로 묶음
// 변하지 않는 속성 Font 캐시 처리
Character c1 = new Character('h', "white", fontFactory.getFont("nanum:12"));
Character c2 = new Character('e', "white", fontFactory.getFont("nanum:12"));
Character c3 = new Character('l', "white", fontFactory.getFont("nanum:12"));
}
}
자주 변하지 않는 속성을 Font 객체로 분리하여 객체를 캐싱한다.
반응형
'Coding > Java' 카테고리의 다른 글
[Java] CompletableFuture 클래스 (Future, CompletionStage) (0) | 2023.12.19 |
---|---|
[Java] 람다 INVOKEDYNAMIC의 내부 동작에 대한 이해 (0) | 2023.04.18 |
wait()과 notify(), notifyAll() (0) | 2022.08.17 |
[JAVA] ThreadLocal (0) | 2022.08.04 |
[JAVA] Volatile 변수 (0) | 2022.08.03 |